An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
JavaScript Array Methods - Filtering
Array.prototype.filter()
I am back again writing about another useful and very common JavaScript (JS) array method and this one does exactly what it says on the tin. It filters. When you have the information stored in an array and you want to pick out (or exclude) specific item(s) that meets a certain condition, .filter()
is your friend.
How to use .filter()
Just like .map()
, .filter()
does not change (mutate) the original array, it creates a new one with all the filtered values. The filter method accepts a function as an argument, and as mentioned before, this is sometimes called callback function (or callback). The callback function should have a return value of true
or false
. You can think of the callback function for filter method as a test for each of the value in the array, if the value passes the test (return true
) then it will be accepted into the new array, otherwise it is cutoff, excommunicado.
You will often see developers writing the callback function as they are calling the .filter()
method, but you can also create the function separately if the test you are performing is extensive, I will show you both in the example below.
Example:
const randomNumbers = [2, 71, 828, 18, 28, 459, 04, 523, 53, 602, 87, 47, 13, 526, 62, 49, 77, 57, 24, 70, 93]function isEven(number) { return number % 2 === 0;}const filteredForEven = randomNumbers.filter(isEven);const filteredForOdd = randomNumbers.filter( number => !isEven(number));console.log(filteredForEven); // [2,828,18,28,4,602,526,62,24,70]console.log(filteredForOdd); // [71,459,523,53,87,47,13,49,77,57,93]console.log(randomNumbers); // [2,71,828,18,28,459,4,523,53,602,87,47,13,526,62,49,77,57,24,70,93]
In the above example, we have an array called randomNumbers
(bonus points if you know where these numbers came from... :D) which we called .filter()
on. I have also defined a function called isEven
to test if the number we pass is even or not.
As you can see, when we filtered the randomNumbers
array for even numbers, we just put the name of the callback function without the parenthesis and the result shows a new array containing just even numbers.
When we filtered for odd numbers, instead of creating a new function to use as a callback function, we wrote the function as an arrow function inside of the .filter()
which says, take the number -> call isEven
with that number -> if it is NOT even then put it in the new array (the exclamation mark ! is JS for NOT).
Summary
Array.prototype.filter():
- Creates a new array by calling the provided callback function on each of the value in your array.
- The callback function must evaluate to
true
orfalse
. - It does not change the original array.
For more detailed information about this method checkout the official documentation on MDN - Mozilla.
If you made it to the end, thank you for reading, if you find any error or have any feedback please leave a comment.
Original Link: https://dev.to/justtanwa/javascript-array-methods-filtering-1k3p

Dev To
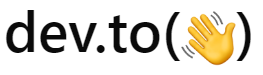
More About this Source Visit Dev To