An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
How to use docker to run a node js application
In this blog, I'll show you how to build a node js application, generate an image for it, and run it using Docker.
Let's create our node js application.
mdkir nodejsappcd nodejsapp
Now lets initialize package.json file using the following command.
npm init
Once your package.json file is created lets now install express.
npm install express
Hence your package.json file looks like this.
{ "name": "nodejsapp", "version": "1.0.0", "description": "nodejsapp description", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "start": "node index.js" }, "author": "", "license": "ISC", "dependencies": { "express": "^4.17.3" }}
Let's get started with index.js
to build our website.
const express = require("express");const app = express();const port=3000;app.get("/", (req, res) => {res.sendFile(__dirname + "/index.html");})app.get("/page", (req, res) => {res.sendFile(__dirname + "/page.html");})app.listen(port, () => { console.log(`running at port ${port}`);});
Let's make two files index.html
and page.html
<!-- index.html --><!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Node JS</title></head><body> <h1>Node JS</h1> <p> Hello from Home </p> <br /> <a href="/page">next page</a></body></html>
<!-- page.html --><!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Node JS Page</title></head><body> <h1>Second Page</h1> <p> Hello from page 2</p> <br /> <a href="/">Home page</a></body></html>
In your root directory, create a Dockerfile
and .dockerignore
.
FROM node:14-alpineRUN mkdir -p /home/appCOPY ./* /home/app/EXPOSE 3000WORKDIR /home/appRUN npm installCMD ["npm", "start"]
node_modules/package-lock.json
To build the docker image run the following command
docker build -t nodeapp:latest .ordocker build -t <dockerhub_name>/<app_name>:<tag> .
To ensure that your Docker image has been built, open a terminal and type docker images
.The output will be displayed as seen below.
REPOSITORY TAG IMAGE ID CREATED SIZEnodeapp latest e0a978b53566 8 seconds ago 123MB
Let us now run our docker image named nodeapp (in my case).
docker run -p 3001:3000 e0a978b53566
Because I have bound my application to port 3001, it runs on that port. You are free to modify it as you see fit.
docker run -p <PORT>:3000 <IMAGE ID>
As a result, you can see your application running http://localhost:3001/
.
I hope this blog is useful to you.
Original Link: https://dev.to/ndrohith09/how-to-use-docker-to-run-a-node-js-application-4ima

Dev To
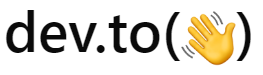
More About this Source Visit Dev To