An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Intersection Observer API
The Intersection Observer API is the browser API that provides an easy way to asynchronously observe the visibility and position of the target element relative to the top-level viewport or the containing root element. Before the introduction of Intersection Observer API, performing such tasks was difficult.
It has several use cases. Some examples among many are lazy-loading of images, infinite scrolling, and animations based on the relative position of elements. The CodePen demo below is the illustration of one such use. CodePen Demo is further explained in the latter part of the blog.
Terminologies
To better understand the Intersection Observer API, we need to be familiar with some of the terminologies used to construct and control the intersection observer.
Root (root)
The root is the element that serves as a viewport to check the visibility of the target element. It must be the ancestor of the target. If unspecified or null, it defaults to the browser viewport.
Root Margin (rootMargin)
It is similar to the CSS margin which serves to grow or shrink the viewport from the top, bottom, left, and right. It can be expressed in pixel and percentage. If unspecified, it defaults to zero.
Intersection Ratio (intersectionRatio)
The degree of intersection between the target element and its root is called the intersection ratio. It is represented as a percentage and has a value between 0.0 to 1.0.
Threshold (threshold)
Threshold is responsible for running the callback function that is passed into the constructor of the intersection observer. Threshold can have a single number or can have an array of numbers to run the callback function. For example, to run the callback function when the visibility of target element inside viewport passes the 30% mark, the value of the threshold is set at 0.3. Similarly, to run a callback function every time the visibility passes 25%, the threshold is set as array [0, 0.25, 0.5, 0.75, 1]. The default, 0 triggers callback function as soon as one pixel is visible in the viewport while the maximum value, 1 means the threshold is not considered passed until every pixel of the target element is visible inside the viewport.
Creating an Intersection Observer
The intersection observer can be created by calling its constructor and passing the callback function and options object. The callback function handles the intersection of the root element and target element while the options object controls all the circumstances at which the callback function is invoked. It has root, rootMargin, and threshold as keys with their respective values.
let options = {
root: document.querySelector('#scrollArea'),
rootMargin: '0px',
threshold: 1.0
}let observer = new IntersectionObserver(callback, options);
let callback = (entries, observer) => {
entries.forEach(entry => {
//do something
});
};
The callback function takes entries and observer as the parameters. "Entries" lists one entry for each target element and represents them as an array. On the other hand, the intersection observer object observes and reports the intersection position of the target elements. Each entry is an instance of IntersectionObserverEntry. IntersectionObserverEntry is an interface of the Intersection Observer API and it describes the intersection between the target element and its root container at a specific moment of transition. It has several useful properties which are briefly discussed below:
boundingClientRect, information about the size of an element and its position relative to the viewport.
intersectionRect, the target visible area.
isIntersecting, if the target element intersects with the root container.
intersectionRatio, the ratio of intersectionRect to the boundingClientRect.
rootBound, position and size of the intersection root.
The target element needs to be selected and observed to report its intersection position.
let target = document.querySelector('#listItem');
observer.observe(target);
To stop observing the target, we can simply useobserver.unobserve(target);
The CodePen demo above utilizes the intersection observer to change the color of the circle by scrolling. The color-changing function and procedure of the circle are controlled by the values of the root, rootMargin, and threshold in the options object. It has three sections. The target element is the middle section with a light green background. The top and bottom sections have light blue backgrounds. The root has the value of null. Thus, the root is the browser viewport. Margin is 0px and threshold is 0 as well. Since, the threshold is 0, as soon as the green background appears in the viewport, the callback function is invoked and the color of the circle changes to green from blue. The color of the circle changes
back to blue once the green background disappears from the viewport.
Conclusion
Intersection Observer API is a powerful tool that can be used to our advantage in several ways in web development. It observes the intersection between the root and target element. The root can be the parent of the target element or the browser viewport. The size of the root can be adjusted using rootMargin. The root has a callback function which is executed when the root crosses the threshold value. Though intersection Observer API observes the target asynchronously, the callback functions are executed synchronously. Lastly, if needed, we can stop observing the target.
Original Link: https://dev.to/syogifse/intersection-observer-api-3nbn

Dev To
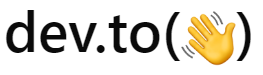
More About this Source Visit Dev To