October 5, 2021 03:15 am GMT
Original Link: https://dev.to/alejomartinez8/how-to-detect-images-loaded-in-react-39fa
How to detect images loaded in React
I had to do a manual deep linking hook, scrolling automatically down in a web application to a specific section, but the delay on images loaded were doing this scroll wrong.
Then, how to detect the loading of the images before executing any action in react? The next hook uses eventListener
with load
and error
events, and detect the HTMLImageElement.complete property of javascript, to determine if all images in a specific wrapper element have been completed.
import { useState, useEffect, RefObject } from "react";export const useOnLoadImages = (ref: RefObject<HTMLElement>) => { const [status, setStatus] = useState(false); useEffect(() => { const updateStatus = (images: HTMLImageElement[]) => { console.log(images.map((image) => image.complete)); setStatus( images.map((image) => image.complete).every((item) => item === true) ); }; if (ref.current) { const imagesLoaded = Array.from(ref.current.querySelectorAll("img")); if (imagesLoaded.length === 0) { setStatus(true); } else { imagesLoaded.forEach((image) => { image.addEventListener("load", () => updateStatus(imagesLoaded), { once: true }); image.addEventListener("error", () => updateStatus(imagesLoaded), { once: true }); }); } } return undefined; }, [ref]); return status;};
Note: is important to add both event load
and error
to avoid any blocking after load pages, in case any image don't load correctly.
To use it you have to pass a ref wrapper to limit the search images.
import { useRef } from "react";import { useOnLoadImages } from "./hooks/useOnLoadImages";import "./styles.css";export default function App() { const wrapperRef = useRef<HTMLDivElement>(null); const imagesLoaded = useOnLoadImages(wrapperRef); return ( <div className="App" ref={wrapperRef}> <h1>Use on Load Images hook</h1> <h2>How to detect images loaded in React</h2> <div> <p>{imagesLoaded && "Images loaded"}</p> <img src="https://i.pinimg.com/564x/8b/09/87/8b09873753b3fede7abc1ffd8a147c2e.jpg" alt="image1" /> <img src="https://i.pinimg.com/564x/39/ed/15/39ed1564db8313300d9759dbbf1e6e2a.jpg" alt="image2" /> <img src="https://i.pinimg.com/564x/bc/ec/24/bcec24fd07fca68fd172d3df5d8b2bb9.jpg" alt="image3" /> </div> </div> );}
Here there a demo Link
Original Link: https://dev.to/alejomartinez8/how-to-detect-images-loaded-in-react-39fa
Share this article:
Tweet
View Full Article

Dev To
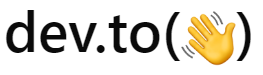
More About this Source Visit Dev To