An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Improve your JS skills with theses tips 2
In this article I will share with you some news tips about JS that can improve your skills!
Don't use delete
to remove property
delete
is very bad to remove a property from an object (bad performance), moreover it will create a lot of side projects.
But what you should do if you need to remove a property?
You need to use Functional approach and create a new object without this property. You can manage to do this with a function like this
const removeProperty = (target, propertyToRemove) => { // We split the property to remove from the target in another object const { [propertyToRemove]: _, ...newTarget } = target return newTarget}const toto = { a: 55, b: 66 }const totoWithoutB = removeProperty(toto, 'b') // { a: 55 }
A very simple snippet that will help you a lot!
Add a property to an object only if it exists
Sometimes we need to add a property to an object if this property is defined. We can make something like this
const toto = { name: 'toto' }const other = { other: 'other' }// The condition is not importantconst condition = trueif (condition) { other.name = toto.name }
It's not very good code anyway...
You can use something more elegant!
// The condition is not importantconst condition = trueconst other = { other: 'other', ...condition && { name: 'toto' }}
If the condition is true, you add the property to your object (it work thanks to the &&
operator)
I could also make this
// The condition is not importantconst condition = trueconst toto = { name: 'toto' }const other = { other: 'other', ...condition && { ...toto }}
Use template literal string
When we learn strings in javascript and we need to concat them with variable we code something like
const toto = 'toto'const message = 'hello from ' + toto + '!' // hello from toto!
It can become garbage if you add other variables and string!
You can use template literal string
You just need to replace simple or double quotes by back-tick.
And wrap all variables by ${variable}
const toto = 'toto'const message = `hello from ${toto}!` // hello from toto!
Short-circuits conditionals
If you have to execute a function just if a condition is true, like
if(condition){ toto()}
You can use a short-circuit just like
condition && toto()
Thanks to the &&
(AND) operator, if the condition is true, it will execute toto function
Set default value for variable
If you need to set a default value to a variable
let totoconsole.log(toto) //undefinedtoto = toto || 'default value'console.log(toto) //default valuetoto = toto || 'new value'console.log(toto) //default value
Thanks to the ||
(OR) operator, if the first value is undefined or false, it will assign the default value after the (||
)!
Use console timer
If you need to know the execution time of a function for example you can console timer. It will give you back the time before and after the execution of your function very fastly!
console.time()for (i = 0; i < 100000; i++) { // some code}console.timeEnd() // x ms
I hope you like this reading!
You can get my new book Underrated skills in javascript, make the difference
for FREE if you follow me on Twitter and MP me
Or get it HERE
You can SUPPORT MY WORKS
You can follow me on
Twitter : https://twitter.com/code__oz
Github: https://github.com/Code-Oz
And you can mark this article!
Original Link: https://dev.to/codeoz/improve-your-js-skills-with-theses-tips-2-3bg2

Dev To
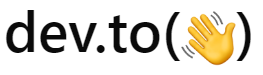
More About this Source Visit Dev To