An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
July 15, 2021 12:34 am GMT
Original Link: https://dev.to/eduardojuliao/interface-segregation-principle-898
Interface Segregation Principle
The Interface Segregation Principle (ISP) states:
A client should not implement interfaces that are not necessary for the application.
In other words, it's preferably that instead of one big interface, the developer creates smaller more manageable interfaces.
Let's say we're tasked to create a game about robots, at first, we design our interface:
public interface IRobot{ string Name { get; set; } void Walk(); void Fly(); void Attack();}
Perfect! We created our contract for what makes a robot... that violates the Interface Segregation Principle, because not all robots can Walk
, Fly
or Attack
.
public class FriendlyRobot : IRobot{ public string Name { get; set; } public void Walk() { // Code } public void Fly() { Console.WriteLine("This robot can't fly!"); } public void Attack() { Console.WriteLine("This robot can't attack!"); }}
To solve this issue, we need to break down into smaller interfaces:
public interface IRobot{ string Name { get; set; }}public interface IWalkable{ void Walk();}public interface IFlyable{ void Fly();}public interface IAggressive{ void Attack();}
Now we can create a Robot the way we want, without implementing extra code that's not necessary, so our FriendlyRobot
will be implemented in a different way:
public class FriendlyRobot : IRobot, IWalkable{ public string Name { get; set; } public void Walk() { // Code }}
Original Link: https://dev.to/eduardojuliao/interface-segregation-principle-898
Share this article:
Tweet
View Full Article

Dev To
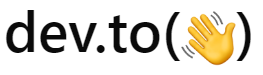
More About this Source Visit Dev To