An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
Build a Chatbot with vanilla JavaScript
Recently, I visited a website and while surfing through it, the websites chatbot caught my attention. I had been looking for a new project to work on, so I got to researching how I could build a chatbot using vanilla JavaScript. While building this project from scratch I stumbled across a lot of difficulties based on the knowledge I had and when I say 'from scratch', I just mean I did not use any additional libraries or APIs while building. Part of this code is based on existing blog posts, articles, and YouTube videos. Also note that this project is more of an insight into JavaScript fundamentals, not any form of artificial intelligence (AI) or machine learning. The main prerequisite for understanding this article is the knowledge of HTML, CSS, and vanilla JavaScript.
Getting Started
Lets create a folder to house our project files, broadly divided into three parts HTML, CSS and JavaScript. Then we build a barebone HTML file which contains all our HTML components:
// index.html<!DOCTYPE html><html lang="en"><head> <title>Chatbot</title> <link rel="stylesheet" href="style.css" /> <script type="text/javascript" src="index.js" ></script></head><body> <h1>Chatbot</h1> <div id="container" class="container"> <input id="input" type="text" placeholder="Say something..." autocomplete="off" /> </div></body></html>
Well do the same for our style.css
file to add styling to our application:
* { box-sizing: border-box; } html { height: 100%; } body { font-family: 'Poppins', sans-serif; background-color: #fff; height: 100%; margin: 0; } .container { width: 100%; height: 100%; }
Listening for events
First off, well have to check if the content of our page has loaded before our script has a chance to run and well also need a keycode to send a message to the chatbot using our enter
key.
For this to work, an addEventListener
method is needed. It calls up a function whenever a specified event is delivered to the target. The two events our addEventListener
listens for are:
DOMContentLoaded
- this event fires when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading.keydown
- this event is fired for all keys, regardless of whether they produce a character value.
KeyboardEvent code
The keydown
event is a KeyboardEvent.code
property which provides a code indicating which of the users keyboard keys is pressed. For example, a lowercase "a" will be reported as 65 by keydown
and keyup
. An uppercase "A" is reported as 65 by both events.
With the help of our keydown
event, we can create an effective way of sending a message to the chatbot by pressing the enter
key. Our addEventListener
would listen and respond anytime the enter
key is pressed.
document.addEventListener("DOMContentLoaded", () => {inputValue.addEventListener("keydown", (e) => { if (e.code === "Enter") { let input = inputValue.value; inputValue.value = ""; output(input); } }); });
In the code snippet above, e.code === "Enter"
indicates the Keycode 13 directly assigned to the Enter
button. To know more about Keycodes, read up on the KeyboardEvent
object.
The input value from the user is assigned to a variable which well make use of later on. One last thing to have in mind is to clear or reset our input once our message is sent, .value = ""
makes this possible. We can use .reset()
if our input field was a form
tag but sadly, it isnt.
Creating User and Chatbot responses
Editing user text input
Next, well create a function for our chatbot behaviour.
function output(input) { //remove all characters except word characters, space, and digits let text = input.toLowerCase().replace(/[^\w\s]/gi, "").replace(/[\d]/gi, "").trim(); text = text .replace(/ a /g, " ") // replaces 'tell me a story' to 'tell me story' .replace(/i feel /g, "") .replace(/whats/g, "what is") // replaces "whats" to "what is" .replace(/please /g, "") .replace(/ please/g, "") .replace(/r u/g, "are you"); //replaces "r u" to "are you"}
Our users input value need to undergo some changes for our chatbot to understand the message sent by the user as shown above and by doing that well have to apply some JavaScript methods which are:
- toLowerCase() - Converting the input values to lowercase.
- Regex and replace() - This removes a non word/space character and digit. For example it replaces certain things like
whats up
towhat is up
orr u
toare you
. If the user sayswhat is going on
,whats going on
, orwhat's going on
, they will all lead to the same valid bot response. - trim() - To trim trailing whitespaces.
Creating a set of arrays
Now that weve gotten a good idea of how the users text input would turn out, well have to create a set of arrays which include possible user texts and another array of appropriate chatbot responses.
const userTexts = [ //0 ["hi", "hey", "hello", "good morning", "good afternoon", "good day"], //1 ["how are you", "how is life", "how are things", "how are you doing", "are you doing good", "are you fine", "how is your day going", "how is your day", "what's up", "whats up", "you good"], //2 ["what are you doing", "what is going on", "what is up", "how is your day", "what's up", "whats up", "you good"], //3 ["how old are you", "are you old"], //4 ["who are you", "are you human", "are you bot", "are you human or bot"], //5 ["who created you", "who made you", "were you created"] ] const botReplies = [ //0 ["Hello!", "Hi!", "Hey!", "Hi there!","Howdy"], //1 [ "Fine... and you?", "Pretty well, and you?", "Fantastic, and you?" ], //2 [ "Nothing much", "About to go to sleep", "Can you guess?", "I don't know actually" ], //3 ["I am infinite"], //4 ["I am just a bot", "I am a bot. What are you?"], //5 ["The one true God, JavaScript"] ]
Well need to create an alternate set of arrays for a situation where the chatbot can not understand the message being sent by the user.
const alternative = [ "Same", "Go on...", "Bro...", "Try again", "I'm listening...", "I don't understand :/" ]
NOTE: You can add extra user texts and reponses if needed.
Compare and match User and Chatbot responses
Our chatbot function still needs an IF/ELSE
statement to compare and match our arrays for a suitable reply or produce an alternate reply if we get a user input that does not match our userTexts
array.
function output(input) { if (compare(userTexts, botReplies, text)) { // search for exact match in `userTexts` finalResult = compare(userTexts, botReplies, text); } else { // if everything else fails, bot produces a random alternative reply finalResult = alternative[Math.floor(Math.random() * alternative.length)]; } // to update our HTML DOM element addToChat(input, finalResult); }
We have to match the user and chatbot arrays to making it look like a conversation between a user and the chatbot. Notice in the code snippet above, if we get a user input that matches an option at userTexts[0] such as hi or hello, the bot will answer with a corresponding reply from its own set of options from botReplies[0] and so on. Now well add the function that matches these two set of arrays.
function compare(userTexts, botReplies, text) { for (let x = 0; x < userTexts.length; x++) { for (let y = 0; y < botReplies.length; y++){ if (userTexts[x][y] == text) { let replies = botReplies[x]; let reply = replies[Math.floor(Math.random() * replies.length)]; } } } return reply; }
The function works like this, well first have to loop through the index of the userTexts array, then well apply another loop to check if our users input text matches any of the responses at that particular index. After checking to see if it matches, well randomly pick a corresponding reply from the set of botReplies arrays available.
Updating our DOM element
Finally well update our HTML DOM (Document Object Model) so our messages can actually display for whenever the user or the chatbot sends a message. Making use of the .appendChild
method, we could create a thread of messages by updating the user and chatbot field every time a message is sent.
function addToChat(input, finalResult) { let userDiv = document.createElement("div"); userDiv.id = "user"; userDiv.className = "response"; userDiv.innerHTML = `<span>${input}</span> messagesContainer.appendChild(userDiv)`; let botDiv = document.createElement("div"); let botImg = document.createElement("img"); let botText = document.createElement("span"); botDiv.id = "bot"; botImg.className = "avatar"; botDiv.className = "bot response"; botText.innerText = "Typing..."; botDiv.appendChild(botImg); botDiv.appendChild(botText); messagesContainer.appendChild(botDiv);}
Video
Click on the image above to watch a demo of our application being tested
Conclusion
By following the steps in this article, you can build a chatbot with plain JavaScript.
For better understanding and overview of the code base of this article, you can check it out on Github.
Original Link: https://dev.to/ugwutotheeshoes/build-a-chatbot-with-vanilla-javascript-5akn

Dev To
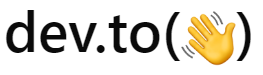
More About this Source Visit Dev To