An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
Solution: Minimum Moves to Equal Array Elements II
This is part of a series of Leetcode solution explanations (index). If you liked this solution or found it useful, please like this post and/or upvote my solution post on Leetcode's forums.
Leetcode Problem #462 (Medium): Minimum Moves to Equal Array Elements II
Description:
(Jump to: Solution Idea || Code: JavaScript | Python | Java | C++)
Given an integer array
nums
of sizen
, return the minimum number of moves required to make all array elements equal.In one move, you can increment or decrement an element of the array by
1
.
Examples:
Example 1: Input: nums = [1,2,3] Output: 2 Explanation: Only two moves are needed (remember each move increments or decrements one element):
[1,2,3] => [2,2,3] => [2,2,2]
Example 2: Input: nums = [1,10,2,9] Output: 16
Constraints:
n == nums.length
1 <= nums.length <= 10^5
-10^9 <= nums[i] <= 10^9
Idea:
(Jump to: Problem Description || Code: JavaScript | Python | Java | C++)
This problem is deceptive in its simplicity. Ultimately, the value to which you want to set each element equal is the median of the sorted nums array. To come to this realization, we have to first think about the nature of the problem.
Let's consider a possible scenario in which we've decided that our target value is x which would take ans number of moves to complete. What would happen to ans if we increased x by 1? If we did, each element that is below the new x would have to spend another move to get up to x, but every element that is above the new x would have to spend one less move to get down to x.
This means that x should naturally move up if there are more elements above x than below. It also means the inverse, that x should move down if there are more elements below x than above. The natural outcome of this is that x will settle at a spot where there are the same number of elements on either side, which is the median value of nums.
To find the median value, we'll have to first sort nums. If nums has an even number of elements, any value between the two middle elements, inclusive, will work for calculating the answer, so we don't have to worry about which of the two elements we use for our solution.
After we have the median value, we can just iterate through nums and find the sum of the differences of each number from the median value, which should be our answer.
- Time Complexity: O(N * log N) where N is the length of nums, for sorting nums
- Space Complexity: O(1)
Javascript Code:
(Jump to: Problem Description || Solution Idea)
var minMoves2 = function(nums) { nums.sort((a,b) => a - b) let ans = 0, median = nums[~~(nums.length / 2)] for (let i = 0; i < nums.length; i++) ans += Math.abs(median - nums[i]) return ans}
Python Code:
(Jump to: Problem Description || Solution Idea)
class Solution: def minMoves2(self, nums: List[int]) -> int: nums.sort() ans, median = 0, nums[len(nums) // 2] for num in nums: ans += abs(median - num) return ans
Java Code:
(Jump to: Problem Description || Solution Idea)
class Solution { public int minMoves2(int[] nums) { Arrays.sort(nums); int ans = 0, median = nums[~~(nums.length / 2)]; for (int num : nums) ans += Math.abs(median - num); return ans; }}
C++ Code:
(Jump to: Problem Description || Solution Idea)
class Solution {public: int minMoves2(vector<int>& nums) { sort(nums.begin(), nums.end()); int ans = 0, median = nums[~~(nums.size() / 2)]; for (auto num : nums) ans += abs(median - num); return ans; }};
Original Link: https://dev.to/seanpgallivan/solution-minimum-moves-to-equal-array-elements-ii-oej

Dev To
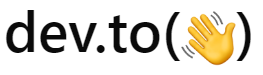
More About this Source Visit Dev To