Some mistakes you may make in PHP
Fast, flexible and pragmatic, PHP powers everything from your blog to the most popular websites in the world. Still during my work, I found it can have tricky behaviors that you may not aware of (yet) and create bugs in your code.
Here are some of the most common mistakes I encountered :
array_merge
Syntax : array_merge ( array ...$arrays ) : array
The function simply merges the elements of arrays together. But it also reset the keys of elements if it's numeric.
For example, a very basic use-case where you have arrays storing [UserId => UserName]
:
$userArr1 = [1 => "John", "3" => "Mary"];$userArr2 = [6 => "Ted", "22" => "Doe"];$allUser = array_merge($userArr1, $userArr2);// $allUser will be// Array// (// [0] => John// [1] => Mary// [2] => Ted// [3] => Doe// )
Casting the numeric value to string like (string)10 won't work. This behavior is same for array_merge_recursive()
, so you should keep this in mind when calling these functions.
empty
Syntax : empty ( mixed $var ) : bool
This check whether a variable is empty. Many use this function to check whether a key is set in array. It's all good and simple until value 0 came in.
For example, an user submit formed validation code :
// Function to check all required field are provided by userfunction checkRequiredFields ($input) { $requiredField = ['name', 'age', 'number_of_kids']; $errors = ''; foreach ($requiredField as $field) { if (empty($input[$field])) { $errors.= "$field is missing"; } } return $errors;}// A valid use-case in real likfe$userData = ['name' => 'John', 'age' => 22, 'number_of_kids' => 0];$inputError = checkRequiredFields($userData);// This return "number_of_kids is missing"
This is because 0 is also considered as "empty" even though it's a valid response in many use-cases. I find people make this mistake mostly when validating user's input.
A work around is adding strlen($var)
!isset($input[$field]) || strlen($input[$field]) == 0
Ternary operator (?:) vs null coalescing operator (??)
When your first argument is null, they're basically the same
$a = null;print $a ?? 'default'; // defaultprint $a ?: 'default'; // default$b = ['a' => null];print $b['a'] ?? 'default'; // defaultprint $b['a'] ?: 'default'; // default
Except that the Ternary operator will output an E_NOTICE when you have an undefined variable.
But when the first argument is not null, they are different :
$a = false ?? 'default'; // false$b = false ?: 'default'; // default
That's it.
Hope my short article can save you some debugging time.
Original Link: https://dev.to/tdx/some-mistakes-you-may-make-in-php-3ld1

Dev To
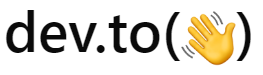
More About this Source Visit Dev To