An Interest In:
Web News this Week
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
- April 19, 2024
- April 18, 2024
- April 17, 2024
Learn React in Plain English
React is a declarative, efficient, and flexible JavaScript library for building user interfaces. It lets you compose complex UIs from small, isolated and reusable pieces of code which are called components.
You can install and use React in two major ways:
- Using npm to install create-react-app
- Manually download React.js and ReactDOM.js files from their website and including it in the script tags of your HTML page.
Create React App is a React code generator which creates scaffolding for your project. With it, you won't have to do much manual configurations and set-up. Under the hood, it uses Babel and webpack, so you really dont need to know anything about them.
Thank God. Webpack isn't really fun to me
The main use of babel is to convert or compile JSX into native JavaScript code.
What is JSX? Dont worry. Were coming to that.
When youre ready to deploy to production, running npm run build will create an optimized build of your app in the build folder. Running this command set's up your development environment:
npx create-react-app test-appcd test-appnpm start
React components are defined as classes. If you're coming from an ES6 background, you should know what classes are.
Classes are simply blueprints for objects. To illustrate, you can think of an architectural plan for a building as a class, and the final building gotten from that plan as an object. The architectural plan won't have properties such as its color, kind of furniture to be used etc. All of those properties will be supplied when "constructing" the building, which is the object.
I hope that made sense? Lol.
When you then instantiate a class by calling its constructor method, you "construct" an object.
When you define a React component, you use the ES6 Class syntax.
If you want a detailed tutorial on ES6 JavaScript, which includes lessons on the "this" keyword you'll see in the below code block, consider buying my Web Development Beginners Guide eBook . I included You Don't know JS - ES6 and beyond for free!
class ProfileDetails extends React.Component { constructor (props) { this.state = { occupation: "student" } } render() { return ( <div className="profile"> <h1>Profile for {this.props.name}</h1> <ul> <li>Male</li> <li>{{this.props.age}}</li> <li>{{this.state.occupation}}</li> </ul> </div> ); }}
Let me break down that code snippet fellas.
- ProfileDetails is the name of this component
- The "extends" keywords indicates that you're extending the parent React Component to build this component. This gives you access to certain functionalities from React.
- The constructor method is where you define what is going to happen when you instantiate this class. This happens when you register this element in a parent element. In our case, we inherit the properties passed from the parent component with the "props" argument. We're also setting a local state data. Don't know what state is? We'll get to that soon. The "this" there is a reference to this current class.
- The props? Well get into that very soon as well.
- The render method is a very important method. It returns the entire markup for this component. Without this, the view part of a component cannot be displayed.
Finally, you noticed some XML-like syntax in the code. Well guess what, that isnt HTML. It is JSX.
JSX is a JavaScript extension created with the aim of simplifying the process of creating elements in React.
Without JSX, this is how you would typically create a React element:
React.createElement("div",null,React.createElement(HelloWorld, null), React.createElement("br", null), React.createElement( "a", { href: "ubahthebuilder.tech" }, "Great JS Resources" ) )
Thanks to JSX, its like you are writing HTML inside of React:
<div> <HelloWorld/> <br/> <a href=" ubahthebuilder.tech ">Great JS Resources</a> </div>
Thats why JSX is useful!
Say you have a "myForm" component class, it's render method should have elements like and other relevant elements because those will constitute the view of "MyForm".
In render(), You can return any set of W3C elements, such as divs, spans, h1 etc, as well as other custom react components.
REACT PROPS
In HTML, when passing a property to an element, you do something like this:
div class="main"
When you're passing Properties to a React element, you should always remember that you can ONLY pass properties from the context of a parent element, which is also the point in which you're registering/instantiating the child element.
For example:
// Parent Classclass App extends React.Component { render() { return ( <div className="profileSection"> <ProfileDetails name="Kingsley" age=21 /> // Child Component Instantaited </div> ); }}
The code snippets are self-explanatory. You only pass in properties (name and age) to the child element (ProfileDetails) from the parent (App). The child class then accesses these properties by using the this.props.NAME syntax.
N/B: A class becomes an element when it gets instantiated by a parent.
Just like elements, you can pass in any of the W3C properties such as title, width, etc as well as define your own custom properties.
STATES
Another way you can pass data around a React component is with states. States, like Props, are also attributes of a React element. The main difference between a Props and State is that while state is defined inside the class in question, Props can only be passed from a parent element.
class Clock extends React.Component { constructor(props) { super(props); this.state = {date: new Date()}; } render() { return ( <div> <h1>Hello, world!</h1> <h2>It is {this.state.date.toLocaleTimeString()}.</h2> </div> ); }}
COMPONENT LIFECYCLE METHODS
The typical lifecycle of a human being is birth, pregnancy, infancy, the toddler years, childhood, puberty, older adolescence, adulthood, middle age, and the matured years.
Well guess what? Components also have lifecycles. They can be broadly classified into these three:
MOUNTING (BIRTH): When any component is created and inserted into DOM(which happens when you call reactDOM.render()), some lifecycle methods used are:
- constructor()
- static getDerivedStateFromProps()
- render()
- componentDidMount()
UPDATING (GROWTH/CHANGE): Any changes to the attributes (Props and State) supplied to a component is will trigger a rerender of that Component, which is also known as the updating phase. In this phase, the following lifecycle methods which get called
- static getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
UNMOUNTED (DEATH): This method is called during the unmounting/destruction of any component:-
- component willUnmount()
COMMON ONES IN DETAIL
constructor(): This lifecycle method runs during the mounting phase of a component. When defining the constructor method, it's very important you inherit Props by using super(props), before any other statements. This method is also the place to define a local state object for the Component. You should not modify the state directly from here, any update has to be done with the setState method.
render(): this method returns the components view in JSX, which is a JavaScript extension similar to HTML. When the parents render method gets called, the action also triggers those of the children components. The parent's render call is complete only after those of it's children's. A rerender (subsequent renders) is triggered whenever any component is updated.
componentDidMount(): This runs after the component is successfully mounted to the DOM. Here, the DOM (Document Object Model) has been painted and is available for further manipulations. This is an apt place to call the setState method.
In addition, any API calls and browser interaction can be made here as well. It is also important to note that this method will run only once, when the component is created.- shouldComponentUpdate(): In this method you can return a Boolean value that specifies whether React should continue with rerendering the UI or not.The default value is true. However, you can specify false or an expression that evaluates to false.
render(): This is the subsequent render mentioned earlier. This happens after the UI Component has received new Props or State.
getSnapshotBeforeUpdate(): As its name implies, This method has access to both the Props and State data just before rerendering/update.
componentDidUpdate(): Very similar to componentDidMount(), this method is called after the component is updated in the DOM.
component willUnmount(): It works as the clean up for any element constructed during componentWillMount.
N/B: If you really want to go into React in-depth, get the HTML to React Course by SleeplessYogi . It's really good!
React is by far one of the most popular and widely-used JavaScript UI library and it is important for web developers to learn (or an alternative) it if they are to build websites professionally.
I will be creating more articles around React, including React Hooks. So make sure you follow this blog and stay updated.
Thank you for reading.
Original Link: https://dev.to/ubahthebuilder/learn-react-in-plain-english-3php

Dev To
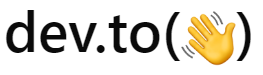
More About this Source Visit Dev To