An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Events and Event Emitter in Node.js
One of the core concepts of a node is the concept of events. In fact, a lot of nodes' core functionality is based on this concept of events.
Definition:
The event is basically a signal that indicates that something has happened in our applications.
Example :
In node, we have a class called HTTP that we can use to build a web server so we listen on a given port, and every time we receive a request on that port that HTTP class raises an event. Now our job is to respond to that event which basically involves reading that request and returning the right response.
Let's see how we can work with the event emitter:
Step-1:
let's load the events modules :
const EventEmitter = require('events');
Note: In terms of naming the first letter of every word in uppercase this is a convention that indicates that this event emitter is a class, not a function or simple value.
When we call the require
function we get the event emitter class. Now, we need to create an instance of this class. And that can be done with the following piece of code
const emitter = new EventEmitter() ;
This emitter has a bunch of methods use this link for all list of events that offer emitter class.
Even though we have more than ten methods most of the time we use only two of these methods one is emit that we use to raise an event. If you do not know the meaning of the emit: means making noise or produce something in our case we are going to making noise in our application. We are signaling that an event has happened.
emitter.emit('')
We pass an argument that is the name of the event let say messageLogged in the future we are going to extend our loger module and every time we log a message we are going to raise an event called message logged
Now , if we run the following codes nothing is going to append :
const EventEmitter = require('events')const emitter = new EventEmitter();emitter.emit('messageLogged');
because we have raised an event here but nowhere in our application we have registered a listener that is interested in that event.
Listener: is a function that will be called when that event is raised.
Let's register a listener that will be called when the message log event is raised for that we will use the on method and that takes two arguments first one will be the name of the event in our case messageLogged and the second one is a callback function or the actual listener.
const EventEmitter = require('events')const emitter = new EventEmitter();//Register a listener emitter.on('messageLogged',function(){ console.log("Listener is called")});emitter.emit('messageLogged');
Note: The order is important here if you register listener after calling the emit method nothing would have happened because when we call the emit method the emitter iterates over all the registered listeners and calls them synchronously.
This is the basis of raising events and handling them using event emitter class.
Happy Coding ;)
Original Link: https://dev.to/ditikrushna/events-and-event-emitter-in-node-js-2ek5

Dev To
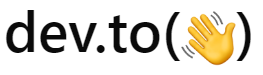
More About this Source Visit Dev To