An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
February 23, 2021 07:45 pm GMT
Original Link: https://dev.to/rickavmaniac/javascript-my-learning-journey-part-5-array-and-object-2aa0
(Javascript) My learning journey Part 5: Array, Object and loop
An essential point to remember a training course is to take notes and discuss the subject with others. That's why every day I will post on dev.to the concepts and notes that I learned the day before.
If you want to miss nothing click follow and you are welcome to comments and discuss with me.
Without further ado here is a summary of my notes for day 5.
Array
An array is a data structure that contains a group of elements. Arrays are commonly used to organize data so that a related set of values can be easily sorted or searched.
// Data store one by onename1 = 'Mike'name2 = 'Paul'name3 = 'John'// More efficiently store in a arraynames = ['Mike', 'Paul', 'John']// Alternative declarationnames = new Array('Mike', 'Paul', 'John')// Accessing a specific array elementconsole.log(names[0]) // Mikeconsole.log(names[1]) // Paulconsole.log(names[2]) // John// Number of entry in arrayconsole.log(names.length)) // 3// Get array last elementconsole.log(names[names.length-1])// Modify a specific elementnames[0] = 'Jack' // replace first entry (Mike) with Jack//
Enter fullscreen mode Exit fullscreen mode
Array basic methods
names = ['Mike', 'Paul', 'John']// Add a element to arraynames.push('Simon') // ['Mike', 'Paul', 'John', 'Simon']// Add a element to the beginning names.unshift('Jack') // ['Jack', 'Mike', 'Paul', 'John', 'Simon']// Remove last elementnames.pop() // ['Jack', 'Mike', 'Paul', 'John']// Remove first elementnames.shift() // ['Mike', 'Paul', 'John']names = ['Mike', 'Paul', 'John']// Find element index positionnames.indexOf('Paul') // 1// If elements includes in arraynames.includes('Paul') // true
Enter fullscreen mode Exit fullscreen mode
Objects
JavaScript objects are containers for named values, called properties and methods.
// Object declarationconst customer = { firstName: 'Mike', // String lastName: 'Tailor', // String emails: ['[email protected]', '[email protected]'], //Array creditLimit: 2500 // Number}// Access object propertyconsole.log(customer.firstName) // Mike// orconsole.log(customer['firstName']) // Mike// Add propertycustomer.phone = "800-828-1240"// orcustomer['phone'] = "800-828-1240"
Enter fullscreen mode Exit fullscreen mode
Objects functions
const customer = { firstName: 'Mike', lastName: 'Tailor', fullName: function() { // this keyword reference the object calling the method return this.firstName + ' ' + this.lastName } }customer.firstName = 'Mike'customer.lastName = 'Taylor'console.log(customer.fullName()) // Mike Taylor// orconsole.log(customer['fullName']() // Mike Taylor
Enter fullscreen mode Exit fullscreen mode
Loop
// For Loop keep running until condition is truefor (let i = 1;i <= 10; i++) { console.log(i)}// For Loop : Break and Continue keywordfor (let i = 1;i <= 10; i++) {if (i === 1) continue // go directly to next interationif (i === 5) break // stop and exit loop console.log(i)}// Loop within a Arraynames = ['Mike', 'Paul', 'John']for (let i = 0;names.length - 1; i++) { console.log(names[i])}// While looplet count = 1while (count <= 10) { count++ console.log(count)}
Enter fullscreen mode Exit fullscreen mode
Conclusion
That's it for part 5. Next day will cover DOM manipulation.
Original Link: https://dev.to/rickavmaniac/javascript-my-learning-journey-part-5-array-and-object-2aa0
Share this article:
Tweet
View Full Article

Dev To
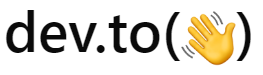
More About this Source Visit Dev To