An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Refactoring checklist for beautiful Ruby code
You should never underestimate the importance of refactoring. If you're new to Ruby, you might be making a detour without knowing how to write shorter code or how to use convenient syntaxes. Since I learned basic Ruby at a coding boot camp, I have been trying to learn from the code of experienced developers and realized that my code was much more verbose and thus confusing. In this article, I will introduce some techniques I found to make Ruby code more readable, less expensive, and easy to change.
Conditional expressions
1. One-line if
statement
# Badif num > 5 puts "#{num} is greater than 5"end
# Goodputs "#{num} is greater than 5" if num > 5
2. Use unless
This might depend on your preference.
# Baduser.destroy if !user.active?
# Gooduser.destroy unless user.active?
3. Ternary operator ?:
# Badif score > 8 result = "Pass"else result = "False"end
# Goodresult = score > 8 ? "Pass" : "False"
4. Avoid == true
, == false
, and == nil
# Badif foo == true # somethingend# Goodif foo # somethingend
# Badif bar == false # somethingend# Goodif !bar # somethingend# orunless bar # somethingend
# Badif baz == nil # somethingend# Goodif !baz # somethingend# orunless baz # somethingend
Or, if you need to check if the value is specifically nil
and not any other falsey value, this might be a better option:
# Goodif baz.nil? # somethingend
5. Safe navigation operator &.
(after Ruby 2.3.0)
The safe navigation operator &.
allows skipping method call when the receiver is nil
.
In the example below, if book.author
or book.author.name
is possibly nil
, you need to check that to avoid NoMethodError
.
# Badif book.author if book.author.name puts "#{book.title} was written by #{book.author.name}" endend
If you use the safe navigation operator, this can be done with fewer lines of code.
# Goodif book.author&.name puts "#{book.title} was written by #{book.author.name}"end
6. Use ||=
for conditional initialization
# Badbook = Book.new if book.nil?
# Goodbook ||= Book.new
Array
7. Use %w()
or %i()
when create an array (Percent strings)
I would say using percent strings is a better way because it will prevent you from forgetting quotation mark or colon and making errors.
# Badgenres = [ "fantasy", "adventure", "mystery", "romance" ]
# Good# Stringgenres = %w(fantasy adventure mystery romance)# =>[ "fantasy", "adventure", "mystery", "romance" ]# Symbolgenres = %i(fantasy adventure mystery romance)# =>[ :fantasy, :adventure, :mystery, :romance ]
8. Use &
(ampersand) operator when iterate over an array
# Badtitles = books.map{ |book| book.title }
# Goodtitles = books.map(&:title)
9. Use index -1
or .last
method to get the last element of an array
genres = [ :fantasy, :adventure, :mystery, :romance ]# Badgenres[genres.length - 1] # => :romance# Goodgenres[-1] # => :romancegenres.last # => :romance
10. Destructuring assignment
Destructuring is a convenient way of extracting multiple values from data stored in objects like arrays.
quotient, remainder = 20.divmod(3) # => [6, 2]quotient # => 6 remainder # => 2
Others
11. Heredoc(Here documents)
If you are writing a large block of text, heredoc will help you make it cleaner and more readable.
# Badtext = "Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
Ut enim ad minim veniam,
quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat."
text = <<-TEXTLorem ipsum dolor sit amet,consectetur adipiscing elit,sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.Ut enim ad minim veniam,quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.TEXT
Hope you find this article helpful and please leave comments if you have any suggestions to add!
Original Link: https://dev.to/junko911/refactoring-checklist-for-beautiful-ruby-code-175c

Dev To
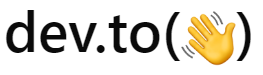
More About this Source Visit Dev To