An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Meet Bower: A Package Manager For The Web
As the web platform has matured, the tools for managing our projects, too, have matured. In this tutorial, I’ll introduce you to one of these tools that makes managing the dependencies of your project considerably easier: Bower.
When I first looked into Bower, I wasn’t exactly sure how it fit in: it wasn’t just a JavaScript package manager, like Jam, and it wasn’t a module loader, like RequireJS. It calls itself a browser package manager, but what exactly does this mean? How’s that different from a JavaScript package manager? The main difference is that Bower doesn’t just handle JavaScript libraries: it will manage any packages, even if that means HTML, CSS, or images. In this case, a package means any encapsulated, third-party code, usually publicly accessible from a Git repository.
Bower is just a package manager.
The important thing to note here is that Bower is just a package manager, and nothing else. It doesn’t offer the ability to concatenate or minify code, it doesn’t support a module system like AMD: it’s sole purpose is to manage packages.
Enough chat: let’s see how this thing works!
Installing Bower
Of course, before we can actually use Bower, we’ll have to install it. This is easy: use NPM:
npm install -g bower
Be sure to install it globally (with -g
), because this is project-specific.
Finding Packages
If you don’t know the name of the package you want, you can find packages by using the bower search
command. If you use it without a search term, you’ll get a list of all the bower packages available. To filter those down, use a search term.
bower searchbower search backbonebower search bootstrap
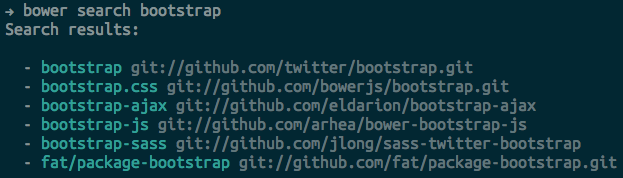
If you’d rather search via a GUI, head over to https://sindresorhus.com/bower-components/.
Installing Packages
Once you’ve found the package you want to install, just use bower install
to install it.
bower install jquerybower install backbone
To install a specific version of a package, include the version number after it, with a hash between:
bower install jquery#1.7.0
Check out that components
folder that Bower just created for us. Inside it, you should see three folders: backbone
, jquery
, and underscore
. Underscore was installed because it’s a dependency of Backbone; that’s handy! Then, if you compare the contents of these folders with their repository URLs (from the search or install output), you’ll see that they are exactly the same. This illustrates well the fact that Bower is, at its most basic level, a short-cut for Git.
But you can pass the bower install
command other things, too. Based on what we’ve just seen, it makes sense that you can use a Git url:
bower install git://github.com/pivotal/jasmine.git
You can also use a link to a single file:
bower install https://backbonejs.org/backbone-min.js
You can even install a package from your own computer: something you’re working on, perhaps:
bower install ~/code/secretProject
You might notice in the output of the bower install
command that it mentions caching the package: it does this by saving a copy of it to ~/.bower/<package>
. Bower can then use this repo to speed up later installations of the same package.
Updating and Uninstalling Packages
If the next version of a library comes out and you’d like to update to it, you can do so easily by running this:
bower update
Note that you can’t pass a package name to the update
command and only update that individual package: it will still update ’em all. You can get the latest version of a single package by running bower install <package>
; it will just over-write the version you currently have.
If your needs ever change, you can easily uninstall a package with the uninstall
command:
bower uninstall jquery
Using Packages
So, we have our packages installed. Now, we want to use them. Remember, Bower is just a package manager, so there’s no Bower-specific way to use these packages. For now, we’ll just stick with regular old script tags:
<div id="test"></div><script src="components/jquery/jquery.js"></script><script> $("#test").text("JQUERY!");</script>
Open this in a browser, and you’ll see the text “JQUERY!”; that’s the sign that it’s working!
As I mentioned above, Bower doesn’t have a system for loading packages onto your page, but that doesn’t mean you shouldn’t use one. Bower is un-opinionated and leaves it up to you to choose the method you’re most comfortable with. Maybe it’s multiple script tags that concatenate and minify at build time. Maybe it’s RequireJS (which we can get, via Bower). Maybe it’s Sprockets or another server side asset packaging system. It’s completely up to you.
Other Bower Commands
There are a few other Bower commands that you should know. First, bower list
or bower ls
will list the packages you currently have installed.
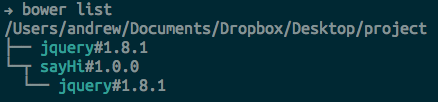
Note that this doesn’t mean we have jQuery installed twice; it means jQuery is a dependency of ’sayHi` (a package we’ll build later on).
If you’d like to see what repository URL a certain package refers to, use the bower lookup
command, using the package name as the argument:
